Introduction
In Excel, navigating through large data sets can be challenging, especially when you need to identify the last row or column of your data.
With VBA (Visual Basic for Applications), you can automate this process to quickly find the last occupied row and column in any worksheet.
In this guide, we’ll show you how to create simple macros that return the last row and last column in Excel, helping you streamline your workflow.
Setting Up Your VBA Environment
Before you start coding, ensure that the Developer tab is enabled in Excel. If you haven’t done so yet, follow these steps:
- Open Excel and click on File.
- Select Options and navigate to Customize Ribbon.
- Check the Developer option and click OK.
Now, the Developer tab will be available on the ribbon, allowing you to access the Visual Basic Editor.
Understanding the Code
We’re going to create two buttons: one to find the last column and another to find the last row in a worksheet. The code will be linked to these buttons, which, when clicked, will display the last row or column in a message box.
Step-by-Step Guide
- Insert a CommandButton to Find the Last Column
First, we need a button to trigger the macro that finds the last column.- Open the Visual Basic Editor (Alt + F11).In the VBA editor, insert a new user form or use an existing worksheet.Add a CommandButton by clicking the Insert tab and selecting CommandButton.
Option Explicit
Private Sub CommandButton1_Click()
Dim Last_Column As Long
Last_Column = Worksheets("Sheet1").Cells(1, Columns.Count).End(xlToLeft).Column
MsgBox Last_Column
End Sub
Explanation
Dim Last_Column As Long
: Declares a variable to store the last column number.Worksheets("Sheet1").Cells(1, Columns.Count).End(xlToLeft).Column
: This line finds the last non-empty column in the first row of “Sheet1” by moving leftward from the very last column.MsgBox Last_Column
: Displays the number of the last column in a message box.
- Insert a CommandButton to Find the Last Row
Similarly, add another button to find the last row in the worksheet
Private Sub CommandButton2_Click()
Dim Last_Row As Long
Last_Row = Worksheets("Sheet1").Cells(Rows.Count, 1).End(xlUp).Row
MsgBox Last_Row
End Sub
Explanation
Dim Last_R
1ow As Long
: Declares a variable to store the last row number.
Worksheets("Sheet1").Cells(Rows.Count, 1).End(xlUp).Row
: Finds the last non-empty row in column A by moving upwards from the bottom of the worksheet.MsgBox Last_Row
: Displays the number of the last row in a message box.
Running the Code
To execute these macros:
- Click on the Developer tab and select Design Mode.
- Double-click on each button to add the macros we just created.
- Exit Design Mode and click the buttons to display the last row or last column in your worksheet.
Full Code
Now that you habe under the step by step code here is the full code
Option Explicit
' Find the last column in the first row
Private Sub CommandButton1_Click()
Dim Last_Column As Long
Last_Column = Worksheets("Sheet1").Cells(1, Columns.Count).End(xlToLeft).Column
MsgBox Last_Column
End Sub
' Find the last row in the first column
Private Sub CommandButton2_Click()
Dim Last_Row As Long
Last_Row = Worksheets("Sheet1").Cells(Rows.Count, 1).End(xlUp).Row
MsgBox Last_Row
End Sub
Flow chart of the code
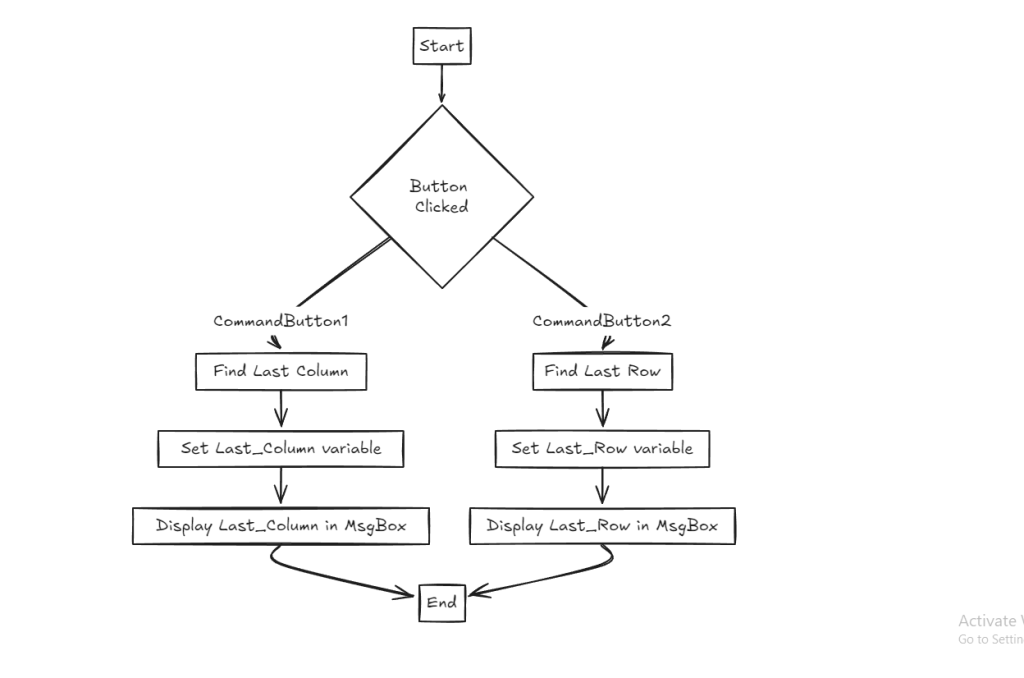
Results
Before Running The Code
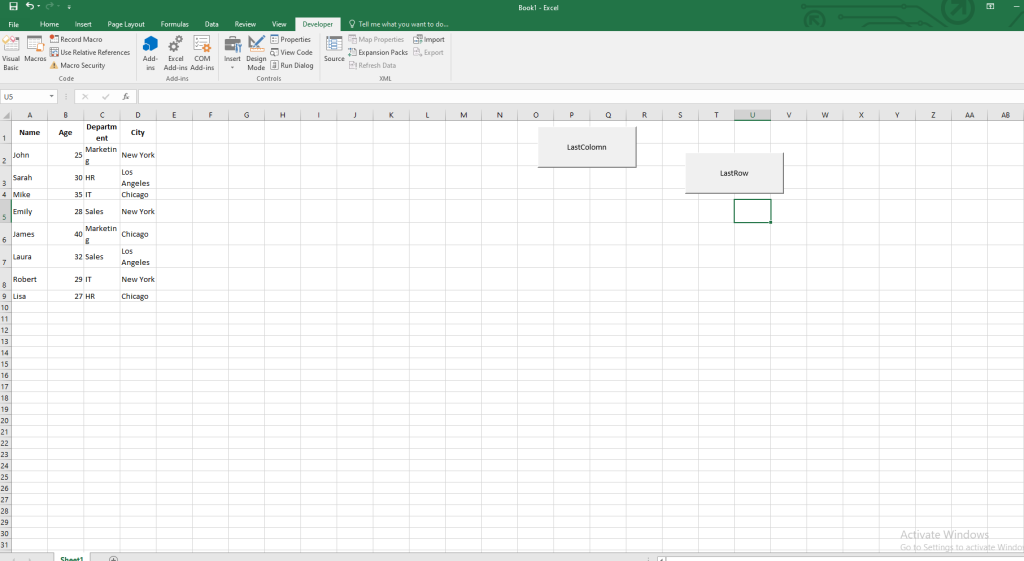
After Running the code for Either Row Or Column
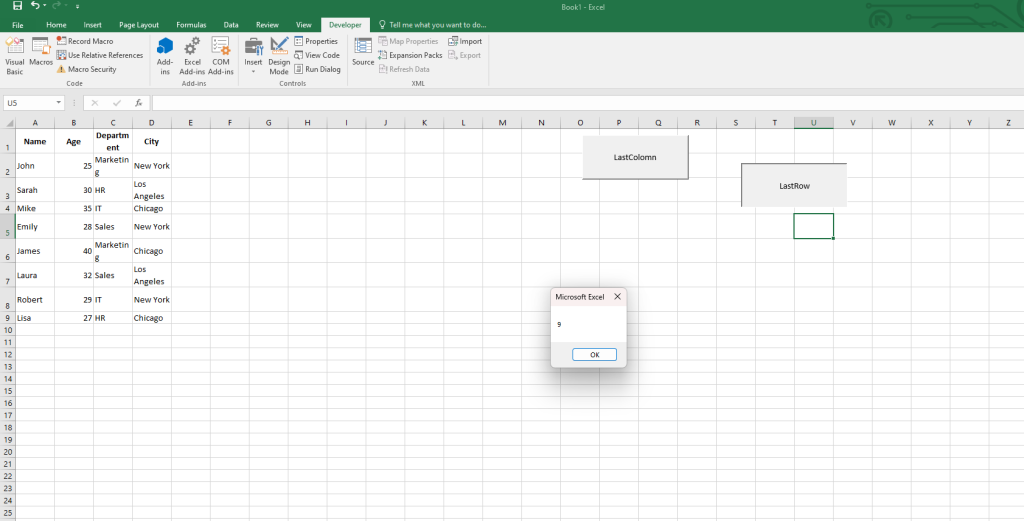
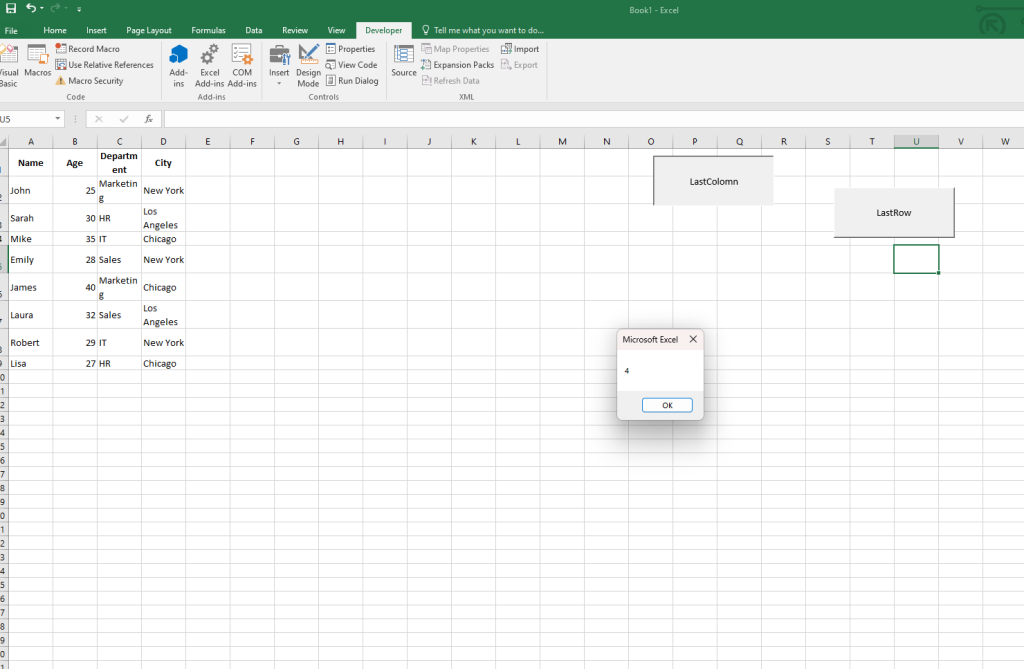
After running the script, a message box will pop up, showing the last column or row in your worksheet. This can be extremely helpful when working with dynamic ranges of data.
Troubleshooting Common Issues
- Ensure you reference the correct sheet name (in this case, “Sheet1”).
- If no message box appears, check if the macro is correctly linked to the button.
Conclusion
By using these simple VBA macros, you can instantly find the last row and column in any Excel worksheet, saving time and making your data management more efficient. Whether you’re a beginner or experienced in VBA, automating tasks like this can improve your productivity in Excel.
Resources
For more information about using VBA in Excel, visit Microsoft’s official VBA documentation. and Pillow
if you like this blog post on How to Find the Last Row and Column in Excel Using VBA, then you would love our other blog post on How to Automatically Organize Data into Separate Sheets Based on Criteria in Excel Using VBA