In this post, I will show how to create a simple organization chart using Python. The NetworkX library can be used to generate and visualize the hierarchy of positions in a company. You will also use matplotlib for plotting.
Setting Up Python on Your Computer
If you don’t have Python installed yet, follow these steps:
- Download the latest version of Python from the official Python website.
- Then Install it by following the instructions for your operating system (Windows, macOS, or Linux).
- And Also Make sure to check the option that says “Add Python to PATH” during installation.
- Then To verify if Python is installed correctly, open your terminal (or command prompt) and type:bashCopy code
python --version
This should display the installed version of Python.
Setting Up Python and Required Libraries
Make sure you have Python installed. Then, run the following to install the required libraries:
pip install networkx numpy matplotlib
Breaking Down the Code
Now, let’s break down the code that creates the organization chart, step by step:
import networkx as nx
import numpy as np
import matplotlib.pyplot as plt
import networkx as nx
: This imports the NetworkX library, used to create and manipulate graphs.import numpy as np
: This imports NumPy, which helps in creating arrays of node numbers.import matplotlib.pyplot as plt
: This imports the plotting library to visualize the chart.
Next, we create a directed graph:
G = nx.DiGraph()
nx.DiGraph()
: This creates a directed graph, ideal for showing hierarchical structures.
Now, we add the nodes and edges to the graph:
nodes = np.arange(0, 8).tolist()
G.add_nodes_from(nodes)
np.arange(0, 8).tolist()
: This generates a list of numbers from 0 to 7, which represent the nodes.G.add_nodes_from(nodes)
: Adds the nodes (positions) to the graph.
We then define the reporting structure through edges:
edges = [(0, 1), (0, 2), (1, 3), (1, 4), (2, 5), (2, 6), (2, 7)]
G.add_edges_from(edges)
edges
: This defines which node (position) reports to another.G.add_edges_from(edges)
: Adds the relationships to the graph.
Next, we define the positions and labels:
pos = {0: (10, 10), 1: (7.5, 7.5), 2: (12.5, 7.5), 3: (6, 6), 4: (9, 6), 5: (11, 6), 6: (14, 6), 7: (17, 6)}
labels = {0: "CEO", 1: "Head of Marketing", 2: "Head of Sales", 3: "Marketing Manager", 4: "Marketing Manager", 5: "Sales Manager", 6: "Sales Manager", 7: "Sales Manager"}
pos
: Specifies the coordinates of each node in the plot.labels
: Assigns job titles to the corresponding nodes.
Finally, we draw the graph and display it:
nx.draw_networkx(G, pos=pos, with_labels=True, labels=labels, arrows=True, node_shape="s", node_size=10000, node_color="skyblue", font_size=10, font_weight="bold", edge_color="gray")
plt.title("Organization Chart")
plt.show()
nx.draw_networkx()
: Renders the graph with the specified positions, labels, and styling.plt.show()
: Displays the chart.
Full Code Example
import networkx as nx
import numpy as np
import matplotlib.pyplot as plt
# Create a directed graph
G = nx.DiGraph()
# Add nodes
nodes = np.arange(0, 8).tolist()
G.add_nodes_from(nodes)
# Add edges
edges = [(0, 1), (0, 2), (1, 3), (1, 4), (2, 5), (2, 6), (2, 7)]
G.add_edges_from(edges)
# Define positions
pos = {0: (10, 10), 1: (7.5, 7.5), 2: (12.5, 7.5), 3: (6, 6), 4: (9, 6), 5: (11, 6), 6: (14, 6), 7: (17, 6)}
# Define labels
labels = {0: "CEO", 1: "Head of Marketing", 2: "Head of Sales", 3: "Marketing Manager", 4: "Marketing Manager", 5: "Sales Manager", 6: "Sales Manager", 7: "Sales Manager"}
# Draw the graph
nx.draw_networkx(G, pos=pos, with_labels=True, labels=labels, arrows=True, node_shape="s", node_size=10000, node_color="skyblue", font_size=10, font_weight="bold", edge_color="gray")
# Display the chart
plt.title("Organization Chart")
plt.show()
Running the Code
To run the code:
- Save the file as
organization_chart.py
. - Run the file in your terminal:bashCopy code
python organization_chart.py
Get access to the script
To make it even easier for you to get started, we’ve uploaded the How to Build an Organization Chart in Python with NetworkX script to our GitHub account. Here’s how you can find and use it:
Visit Our GitHub Repository
- Go to GitHub: Open your web browser and visit our GitHub repository at https://github.com/michealtal/Organisation-chart.git
- Explore the Repository: You’ll see a list of files and folders in the repository. Look for the file named Org_Chart.py
Result
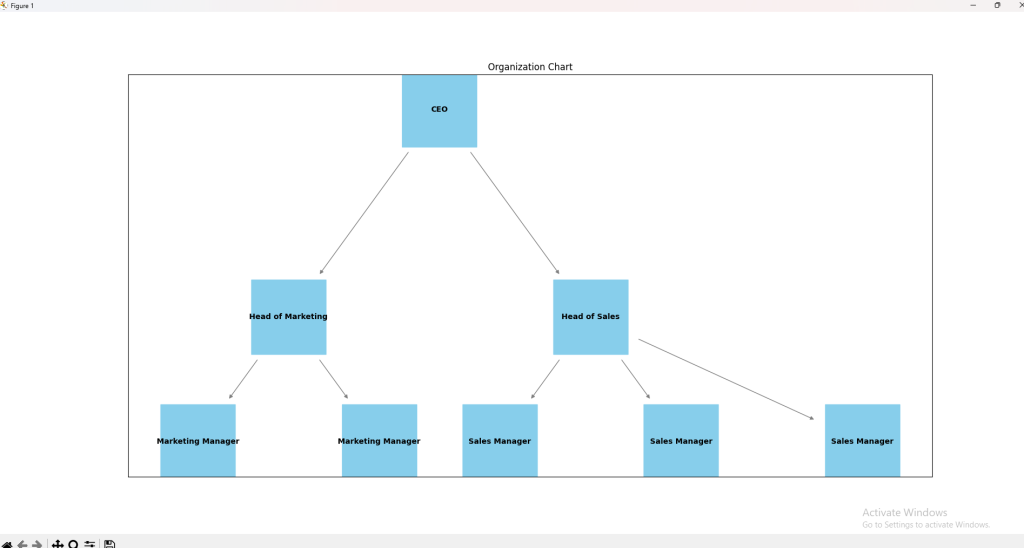
Troubleshooting
- Graph Not Displaying: Ensure you have installed
matplotlib
correctly. Runpip install matplotlib
if needed. - Positions Overlapping: Modify the coordinates in the
pos
dictionary to space the nodes differently. - Node Colors Not Appearing: Ensure
node_color
andnode_shape
are correctly specified in thenx.draw_networkx
function.
Conclusion
This post explains how to create a simple organization chart using NetworkX in Python. The code can be modified to suit your needs by changing the roles or structure. You can also experiment with different node colors and sizes.
For more ideas, check out the NetworkX and Matplotlib documentation.
If you like blog post like this then you would love our other blog post like How to Automate Image Editing in Python: A Beginner’s Guide