Transferring data from Excel to PowerPoint manually can take time Using a VBA macro you can automate this process and move data with one click This guide will show you how to set it up in your Excel workbook
With that been said we would need to set up our enviroment first then to writing of the code so let dive in
Setting Up the Excel VBA Environment
Before running the code, ensure you’ve enabled macros in Excel. This allows VBA scripts to run properly, especially for automation tasks like copying Excel data to PowerPoint.
Breaking Down the Code
Now, let’s break down the code that will help you copy data from Excel and paste it into PowerPoint. Here’s how it works, step by step:
Option Explicit
Sub Button1_Click()
This initializes the Button1_Click
subroutine that will execute when the button is clicked. The Option Explicit
forces you to declare all variables, helping avoid errors due to undeclared variables.
Dim r As Range
Dim powerpointapp As Object
Dim mypresentation As Object
Dim myslide As Object
Dim myshape As Object
This section declares the variables used in the code. The Range
object is used to specify the data range in Excel, and Object
types are used for PowerPoint-related variables.
Set r = ThisWorkbook.Worksheets("sheet1").Range("A1:G11")
Here, the data range to be copied is defined. It targets cells A1 to G11 on “sheet1.”
On Error Resume Next
Set powerpointapp = GetObject(class:="PowerPoint.Application")
If powerpointapp Is Nothing Then Set powerpointapp = CreateObject(class:="Powerpoint.Application")
On Error GoTo 0
This block attempts to connect to an open instance of PowerPoint. If PowerPoint is not open, it creates a new instance.
Set mypresentation = powerpointapp.Presentations.Add
Set myslide = mypresentation.Slides.Add(1, 12)
A new PowerPoint presentation is created, and a blank slide is added to it.
r.Copy
myslide.Shapes.PasteSpecial DataType:=2
The defined Excel range is copied and pasted into the PowerPoint slide. DataType:=2
ensures that the data is pasted as an enhanced metafile for better quality.
Set myshape = myslide.Shapes(myslide.Shapes.Count)
myshape.Left = 250
myshape.Top = 150
This grabs the last shape added to the slide (the pasted data) and sets its position within the slide.
powerpointapp.Visible = True
powerpointapp.Activate
Application.CutCopyMode = False
PowerPoint is made visible, brought to the front, and the clipboard is cleared after the operation.
Full Code Example
Here’s the complete code you can copy and run:
Option Explicit
Sub Button1_Click()
Dim r As Range
Dim powerpointapp As Object
Dim mypresentation As Object
Dim myslide As Object
Dim myshape As Object
Set r = ThisWorkbook.Worksheets("sheet1").Range("A1:G11")
On Error Resume Next
Set powerpointapp = GetObject(class:="PowerPoint.Application")
If powerpointapp Is Nothing Then Set powerpointapp = CreateObject(class:="Powerpoint.Application")
On Error GoTo 0
Set mypresentation = powerpointapp.Presentations.Add
Set myslide = mypresentation.Slides.Add(1, 12)
r.Copy
myslide.Shapes.PasteSpecial DataType:=2
Set myshape = myslide.Shapes(myslide.Shapes.Count)
myshape.Left = 250
myshape.Top = 150
powerpointapp.Visible = True
powerpointapp.Activate
Application.CutCopyMode = False
End Sub
Running the Code
To run this code:
- Open Excel and press
Alt + F11
to open the VBA editor. - Insert a module and paste the code.
- Go back to the Excel sheet and create a button that will trigger the
Button1_Click
macro. - Adjust the data range in the code to match the data you want to copy.
- Press the button to run the code. The data will be copied to a new PowerPoint slide.
Result
Before
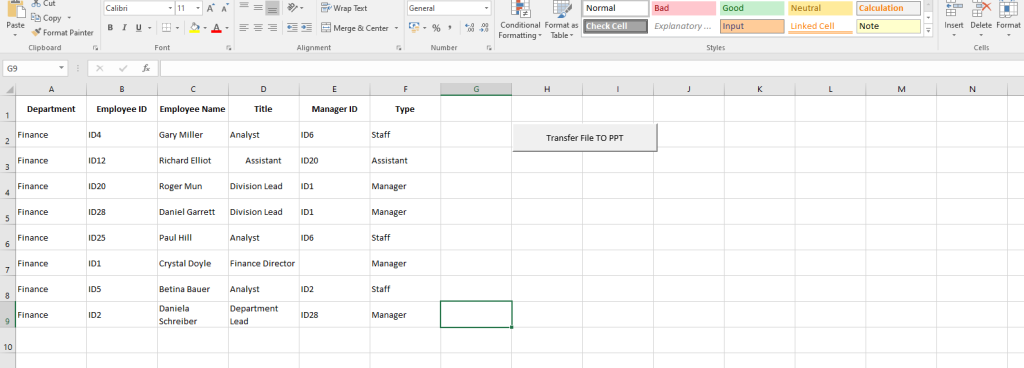
After
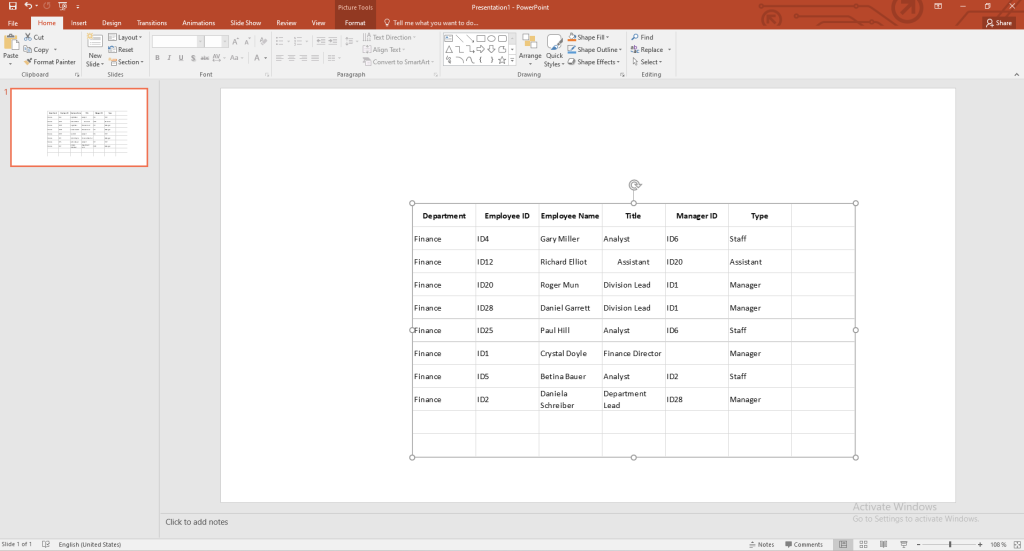
Troubleshooting
- PowerPoint Not Opening: Ensure PowerPoint is installed on your machine. If it’s installed but not opening, check the VBA references in the editor for any missing libraries.
- Incorrect Data Range: Double-check that the range specified in the code matches the actual data in your worksheet.
- Clipboard Issues: If the data isn’t copied correctly, clear the clipboard manually and run the script again.
Code FlowChart
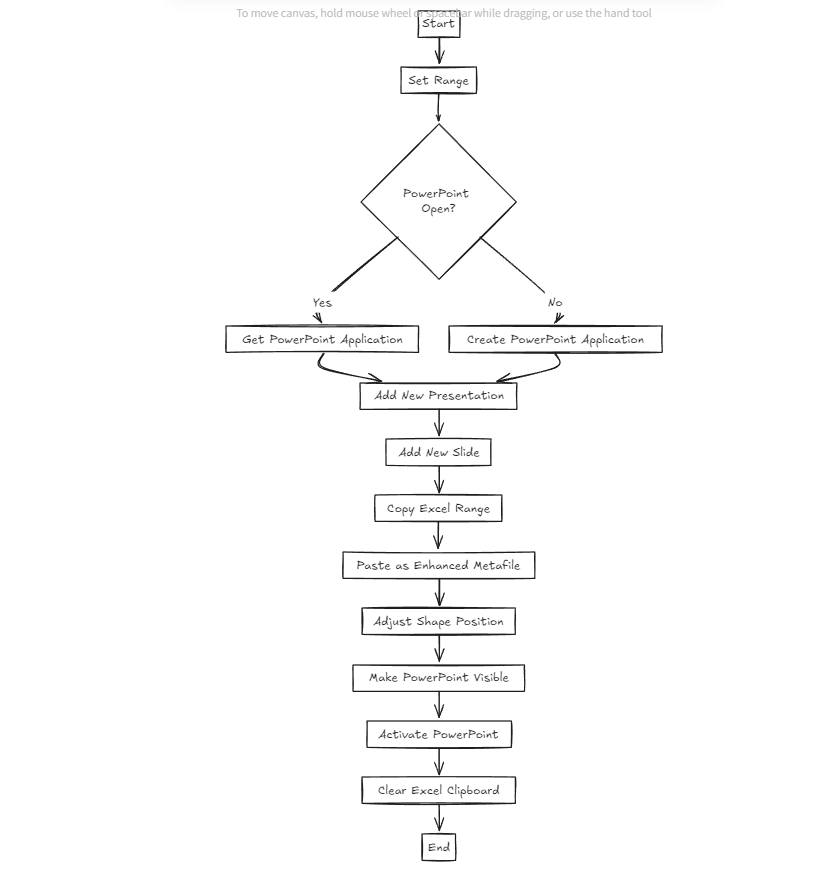
Conculsion
Automating the process of moving data from Excel to PowerPoint with VBA makes your workflow smoother and more efficient Once set up, you can transfer data with a single click, saving time and reducing manual effort This method is useful for anyone working with reports or presentations
If you like blog post like thispost on How to Copy Excel Data to PowerPoint with a Single Button Click Using VBA then you would love our other blog post like How to Automate Image Editing in Python: A Beginner’s Guide