Introduction
Automating data entry in Excel using VBA can save a significant amount of time and reduce errors.
By creating a simple user form with text boxes, option buttons, checkboxes, and list boxes, we can efficiently collect and record data into a worksheet.
In this guide, you’ll learn how to build a basic form, handle user input, and automatically populate the worksheet with the submitted data.
1. Setting Up the Environment
Before we dive into the code, let’s start by creating a new user form in the VBA editor.
- Press
Alt + F11
in Excel to open the VBA Editor. - Insert a new user form by clicking Insert > UserForm.
- Now, add the following controls to the form:
- A TextBox for the user’s name.
- Two OptionButtons for selecting gender.
- Two CheckBoxes for other preferences.
- A ListBox for selecting a favorite sport.
- Two CommandButtons for submitting and closing the form.
2. Writing the VBA Code
Once you’ve set up the form and controls, it’s time to start coding.
Step 1: Handling Text Box and Option Button Input
Here’s how we capture the data entered into the TextBox and OptionButtons and write it into the worksheet.
Private Sub CommandButton1_Click()
Worksheets("Sheet1").Cells(3, 1).Value = TextBox1.Text
- TextBox1.Text: This line takes the value entered into the text box and stores it in cell A3 of Sheet1.
Next, we handle the OptionButtons, which allow the user to select their gender:
If OptionButton1.Value = True Then
Worksheets("Sheet1").Cells(3, 2).Value = "Male"
End If
If OptionButton2.Value = True Then
Worksheets("Sheet1").Cells(3, 2).Value = "Female"
End If
- OptionButton1/OptionButton2: If OptionButton1 is selected, it writes “Male” to cell B3. If OptionButton2 is selected, it writes “Female.”
Step 2: Handling CheckBox Input
We’ll now handle the CheckBoxes that allow the user to select additional options.
If CheckBox1.Value = True Then
Worksheets("Sheet1").Cells(3, 3).Value = "Yes"
End If
If CheckBox2.Value = True Then
Worksheets("Sheet1").Cells(3, 4).Value = "Yes"
End If
- CheckBox1/CheckBox2: If the user selects these checkboxes, it writes “Yes” in columns C and D.
Step 3: Capturing the ListBox Selection
The ListBox allows the user to select their favorite sport. Here’s how we handle that:
Worksheets("sheet1").Cells(3, 5).Value = ListBox1.Value
- ListBox1.Value: This writes the selected sport to cell E3.
Step 4: Closing and Unloading the Form
Finally, we’ll add functionality to close the form when the user clicks the exit button.
Private Sub CommandButton2_Click()
Unload UserForm1
End Sub
- Unload UserForm1: This command closes the form and clears it from memory.
3. Initializing the User Form
To make the user form more dynamic, let’s add some sports options to the ListBox when the form is initialized. This will allow the user to choose from predefined options like Hockey, Soccer, and Basketball.
Private Sub UserForm_Initialize()
ListBox1.AddItem "Hockey"
ListBox1.AddItem "Tennis"
ListBox1.AddItem "Soccer"
ListBox1.AddItem "FootBall"
ListBox1.AddItem "BasketBall"
ListBox1.AddItem "TableTennis"
ListBox1.AddItem "Boxing"
End Sub
- UserForm_Initialize: This event runs when the form is first opened. It populates the ListBox with a list of sports.
4. Full Code
Here is the complete VBA code for your user form:
Option Explicit
Private Sub CommandButton1_Click()
Worksheets("Sheet1").Cells(3, 1).Value = TextBox1.Text
If OptionButton1.Value = True Then
Worksheets("sheet1").Cells(3, 2).Value = "Male"
End If
If OptionButton2.Value = True Then
Worksheets("sheet1").Cells(3, 2).Value = "Female"
End If
If CheckBox1.Value = True Then
Worksheets("sheet1").Cells(3, 3).Value = "Yes"
End If
If CheckBox2.Value = True Then
Worksheets("sheet1").Cells(3, 4).Value = "Yes"
End If
Worksheets("sheet1").Cells(3, 5).Value = ListBox1.Value
End Sub
Private Sub CommandButton2_Click()
Unload UserForm1
End Sub
Private Sub UserForm_Initialize()
ListBox1.AddItem "Hockey"
ListBox1.AddItem "Tennis"
ListBox1.AddItem "Soccer"
ListBox1.AddItem "FootBall"
ListBox1.AddItem "BasketBall"
ListBox1.AddItem "TableTennis"
ListBox1.AddItem "Boxing"
End Sub
5. Running the Script
To run the form:
- Press
F5
in the VBA editor to show the user form. - Enter data in the form and click the submit button.
- The data will automatically be saved into your Excel worksheet in cells A3 to E3.
The flowchart of the code on How to Use VBA to Input Data from a User Form into Excel
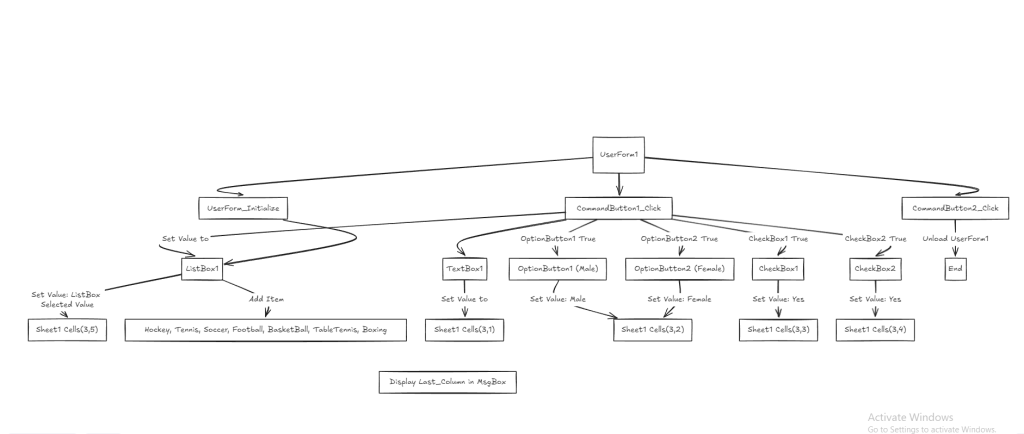
6. Results
Once you run the form, you’ll see the data you entered populate your worksheet in real-time.
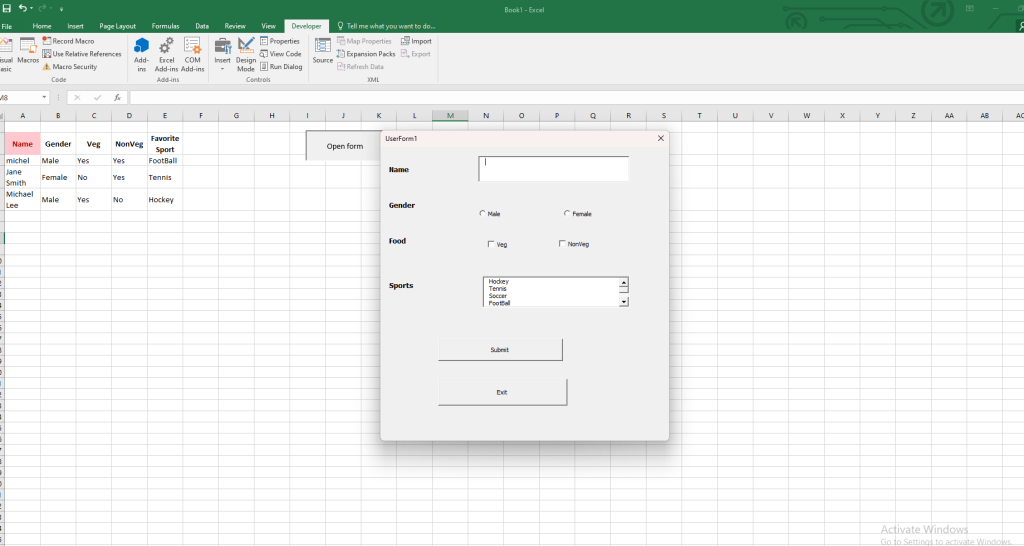
Troubleshooting Common Issues
If you encounter any issues, here are a few things to check:
- Ensure that the form is properly set up: Make sure you have added all the necessary controls (TextBox, OptionButtons, CheckBoxes, ListBox, CommandButtons).
- Check for typos in your code, especially in object names like
TextBox1
,OptionButton1
, etc. - Make sure the worksheet name is correct: If you’re not working with “Sheet1”, update the sheet reference in your code.
Conclusion
Using VBA to automate data entry in Excel is a powerful way to streamline your workflow. In this guide, we’ve walked through creating a simple user form to input data, which is then automatically entered into an Excel sheet. This is just the beginning—once you have this form working, you can extend it to handle more fields, complex validation, and even interactions with external data sources.
Try out this project in your own work and see how VBA can help make Excel even more efficient!
Resources
For more information about using VBA in Excel, visit Microsoft’s official VBA documentation. and Pillow
if you like this blog post on How to Use VBA to Input Data from a User Form into Excel, then you would love our other blog post on How to Sort Data in Excel Using VBA Buttons